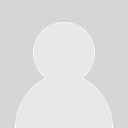
+5
Answered
html interface
Good day, I am really new to EBS enviroments. Is it possible that someone can show me a basic example of how to get a web page interface with a warewolf program. I have currently made a html page to display the rolldice example program with in Warewolf, but cant figure out how to link the two. Any help would be greatly appreciated
Answer

Under review
Hi,
Warewolf is an Enterprise Service Bus that exposes data via micro services. So it is designed to make data available via HTTP (and HTTPS) micro services, both in XML and JSON formats.
What this effectively means is that Warewolf does not serve HTML like a traditional web server, so it cannot display web pages directly. That being said, Warewolf can definitely return JSON or XML data to a page on a web server that contains Javascript to do this.
There is a sample folder in Warewolf program folder that contains a range of samples describing how to connect to Warewolf micro services with various software languages.
To answer your question, the example below describes how to return the dice roll from the Dice Roll microservice on a Warewolf server and display it on a HTML page hosted on a server.
Warewolf is an Enterprise Service Bus that exposes data via micro services. So it is designed to make data available via HTTP (and HTTPS) micro services, both in XML and JSON formats.
What this effectively means is that Warewolf does not serve HTML like a traditional web server, so it cannot display web pages directly. That being said, Warewolf can definitely return JSON or XML data to a page on a web server that contains Javascript to do this.
There is a sample folder in Warewolf program folder that contains a range of samples describing how to connect to Warewolf micro services with various software languages.
To answer your question, the example below describes how to return the dice roll from the Dice Roll microservice on a Warewolf server and display it on a HTML page hosted on a server.
<html><head></head> <html> <body> <h1>Dice Roll Example</h1> <div id="dicediv"></div> <script> var xmlhttp = new XMLHttpRequest(); var url = "http://localhost:3142/services/My%20Category/Dice%20Roll.json?%3CDataList%3E%3C/DataList%3E&wid=28681805-a0df-4bea-8bdc-8afeca830454"; xmlhttp.onreadystatechange=function() { if (xmlhttp.readyState == 4 && xmlhttp.status == 200) { myFunction(xmlhttp.responseText); } } xmlhttp.open("GET", url, true); xmlhttp.send(); function myFunction(response) { var arr = JSON.parse(response); var i; var out = "<table>"; for(i = 0; i < arr.length; i++) { out += "<tr><td>" + "Dice Roll =" + "</td><td>" + arr[i].DiceRoll + "</td></tr>"; } out += "</table>" document.getElementById("dicediv").innerHTML = out; } </script> </body> </html>I hope this helps.
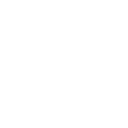
Thank for the Answer it is exactly what i am looking for, however when i run this code i dont get a result print out in the dicediv. When i enter http://localhost:3142/services/My%20Category/Dice%20Roll.json?%3CDataList%3E%3C/DataList%3E&wid=28681805-a0df-4bea-8bdc-8afeca830454 directly into the brower i do however get a json array print out which shows me that Warewolf is running and all is fine on Warewolf's side. Any idea why on why i dont get a print out in the dicediv?

Hi Kyle,
This article :
http://community.warewolf.io/topic/530506-executing-warewolf-service-in-javascript/
might hold the answer. It describes another way to return data from a Warewolf micro service that accounts for security. By default windows runs in a user context, but that gets lost when Javascript calls are made.
Something else that you can look at is the security permissions for both the Warewolf server and the individual microservices. This article describes how to set up permissions.
http://community.warewolf.io/topic/516326-security/
This article describes how to set up public permissions.
http://community.warewolf.io/topic/563251-secuirty-public/
Also, you should really be serving this page via a Web Server. You can run it locally via a browser, but most browsers disable XMLHttpRequest() by default for security reasons. You can however override this in Chrome by starting Chrome in a mode that allows file access from files. like this:
C:\Program Files (x86)\Google\Chrome\Application\chrome.exe --allow-file-access-from-files
This may be acceptable for development environments, but little else. You certainly don't want this on all the time.
This article :
http://community.warewolf.io/topic/530506-executing-warewolf-service-in-javascript/
might hold the answer. It describes another way to return data from a Warewolf micro service that accounts for security. By default windows runs in a user context, but that gets lost when Javascript calls are made.
Something else that you can look at is the security permissions for both the Warewolf server and the individual microservices. This article describes how to set up permissions.
http://community.warewolf.io/topic/516326-security/
This article describes how to set up public permissions.
http://community.warewolf.io/topic/563251-secuirty-public/
Also, you should really be serving this page via a Web Server. You can run it locally via a browser, but most browsers disable XMLHttpRequest() by default for security reasons. You can however override this in Chrome by starting Chrome in a mode that allows file access from files. like this:
C:\Program Files (x86)\Google\Chrome\Application\chrome.exe --allow-file-access-from-files
This may be acceptable for development environments, but little else. You certainly don't want this on all the time.
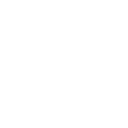
Thank you for all the speedy replies i really appreciate it. I tried all the solutions but to no avail. I will keep working on this until it works. I am interseted in incorporating AngularJS with it but need to get this code to work first.

Hi Kyle,
What json file are you trying to open? Would you mind pasting a copy of how you trying to execute the service?
Thanks
Hagashen
What json file are you trying to open? Would you mind pasting a copy of how you trying to execute the service?
Thanks
Hagashen
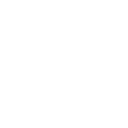
HI Hagashen the code i am trying to run is...
<html><head></head>
<html>
<body>
<h1>Dice Roll Example</h1>
<div id="dicediv"></div>
<script>
var xmlhttp = new XMLHttpRequest();
var url = "http://localhost:3142/services/My%20Category/Dice%20Roll.json?%3CDataList%3E%3C/DataList%3E&wid=28681805-a0df-4bea-8bdc-8afeca830454";
xmlhttp.onreadystatechange=function() {
if (xmlhttp.readyState == 4 && xmlhttp.status == 200) {
myFunction(xmlhttp.responseText);
}
}
xmlhttp.open("GET", url, true);
xmlhttp.send();
function myFunction(response) {
var arr = JSON.parse(response);
var i;
var out = "<table>";
for(i = 0; i < arr.length; i++) {
out += "<tr><td>" +
"Dice Roll =" +
"</td><td>" +
arr[i].DiceRoll +
"</td></tr>";
}
out += "</table>"
document.getElementById("dicediv").innerHTML = out;
}
</script>
</body>
</html>
When i enter ...
http://localhost:3142/services/My%20Category/Dice%20Roll.json?%3CDataList%3E%3C
into my browser i get the output file and i can view the json file itself but when i run all the
code above it doesnt show me anything except the h1 tag i.e. Dice Roll Example. I am
having this problem with another site i am building as well. It allows me to see json files in
same folder but when i try to call json files from local servers or external sites it doesnt display them.
<html><head></head>
<html>
<body>
<h1>Dice Roll Example</h1>
<div id="dicediv"></div>
<script>
var xmlhttp = new XMLHttpRequest();
var url = "http://localhost:3142/services/My%20Category/Dice%20Roll.json?%3CDataList%3E%3C/DataList%3E&wid=28681805-a0df-4bea-8bdc-8afeca830454";
xmlhttp.onreadystatechange=function() {
if (xmlhttp.readyState == 4 && xmlhttp.status == 200) {
myFunction(xmlhttp.responseText);
}
}
xmlhttp.open("GET", url, true);
xmlhttp.send();
function myFunction(response) {
var arr = JSON.parse(response);
var i;
var out = "<table>";
for(i = 0; i < arr.length; i++) {
out += "<tr><td>" +
"Dice Roll =" +
"</td><td>" +
arr[i].DiceRoll +
"</td></tr>";
}
out += "</table>"
document.getElementById("dicediv").innerHTML = out;
}
</script>
</body>
</html>
When i enter ...
http://localhost:3142/services/My%20Category/Dice%20Roll.json?%3CDataList%3E%3C
into my browser i get the output file and i can view the json file itself but when i run all the
code above it doesnt show me anything except the h1 tag i.e. Dice Roll Example. I am
having this problem with another site i am building as well. It allows me to see json files in
same folder but when i try to call json files from local servers or external sites it doesnt display them.

+2
PINNED
Hi Kyle.
Here is a simpler way to get return values back from Warewolf that works:
The one thing I have done is to exclude the <Datalist></Datalist> input variable from the URL as it is not required in this case. I also added an onload reference to the body tag, to run the JavaScript method when the page loads,
If you were using JQuery you could get the method to run when JQuery initializes, but an event needs to be specified to fire the function in this case.
I hope this answers your question.
Here is a simpler way to get return values back from Warewolf that works:
The one thing I have done is to exclude the <Datalist></Datalist> input variable from the URL as it is not required in this case. I also added an onload reference to the body tag, to run the JavaScript method when the page loads,
If you were using JQuery you could get the method to run when JQuery initializes, but an event needs to be specified to fire the function in this case.
I hope this answers your question.
<html><head></head>
<body onload="GetRoll()">
<h1>Dice Roll Example</h1>
<div id="dicediv"></div>
<script type="text/javascript">
//This function demonstrates how to use a standard JasvaScript function to execute a Warewolf workflow.
function GetRoll() {
var xmlhttp = new XMLHttpRequest();
xmlhttp.withCredentials = true; //Required to ensure that Warewolf Server correctly authenticates.
// Note : I am calling Dice%20Roll.json to return JSON
// If I wanted to return XML I would have called Dice%20Roll.xml
xmlhttp.open("GET", "http://localhost:3142/services/My%20Category/Dice%20Roll.json?&wid=28681805-a0df-4bea-8bdc-8afeca830454", true);
xmlhttp.onload = function(e) {
var data = xmlhttp.responseText // The data received from the workflow execution.
callback(data);
}
xmlhttp.send();
};
function callback(data)
{
// See http://www.w3schools.com/json/json_eval.asp for details on the JSON parser
var obj = JSON.parse(data);
document.getElementById("dicediv").innerHTML = "You rolled a " + obj.DiceRoll[0];
};
</script>
</body>
</html>
Customer support service by UserEcho
Here is a simpler way to get return values back from Warewolf that works:
The one thing I have done is to exclude the <Datalist></Datalist> input variable from the URL as it is not required in this case. I also added an onload reference to the body tag, to run the JavaScript method when the page loads,
If you were using JQuery you could get the method to run when JQuery initializes, but an event needs to be specified to fire the function in this case.
I hope this answers your question.